Effect
Parallax Hover Interaction in Framer
This is a code override for Framer that allows any element to follow the cursor at varying intensities. Feel free to remix the project and have fun exploring this effect on your website.



About the resource
This code override can be utilized in various ways, but one fun use case I've discovered is crafting a parallax hover effect.
You can accomplish this by applying the override to several elements with differing intensities. Elements in the foreground will move more rapidly, while those in the background move more slowly.
The code override includes three pre-defined intensity levels.
About the resource
This code override can be utilized in various ways, but one fun use case I've discovered is crafting a parallax hover effect.
You can accomplish this by applying the override to several elements with differing intensities. Elements in the foreground will move more rapidly, while those in the background move more slowly.
The code override includes three pre-defined intensity levels.
About the resource
This code override can be utilized in various ways, but one fun use case I've discovered is crafting a parallax hover effect.
You can accomplish this by applying the override to several elements with differing intensities. Elements in the foreground will move more rapidly, while those in the background move more slowly.
The code override includes three pre-defined intensity levels.

Look override intensities.

Look override intensities.

Look override intensities.
You can also define your own intensities. If you dive into the code override, you can tweak these values found at the bottom of the code to adjust how much the elements follow the cursor.
You can also define your own intensities. If you dive into the code override, you can tweak these values found at the bottom of the code to adjust how much the elements follow the cursor.
You can also define your own intensities. If you dive into the code override, you can tweak these values found at the bottom of the code to adjust how much the elements follow the cursor.
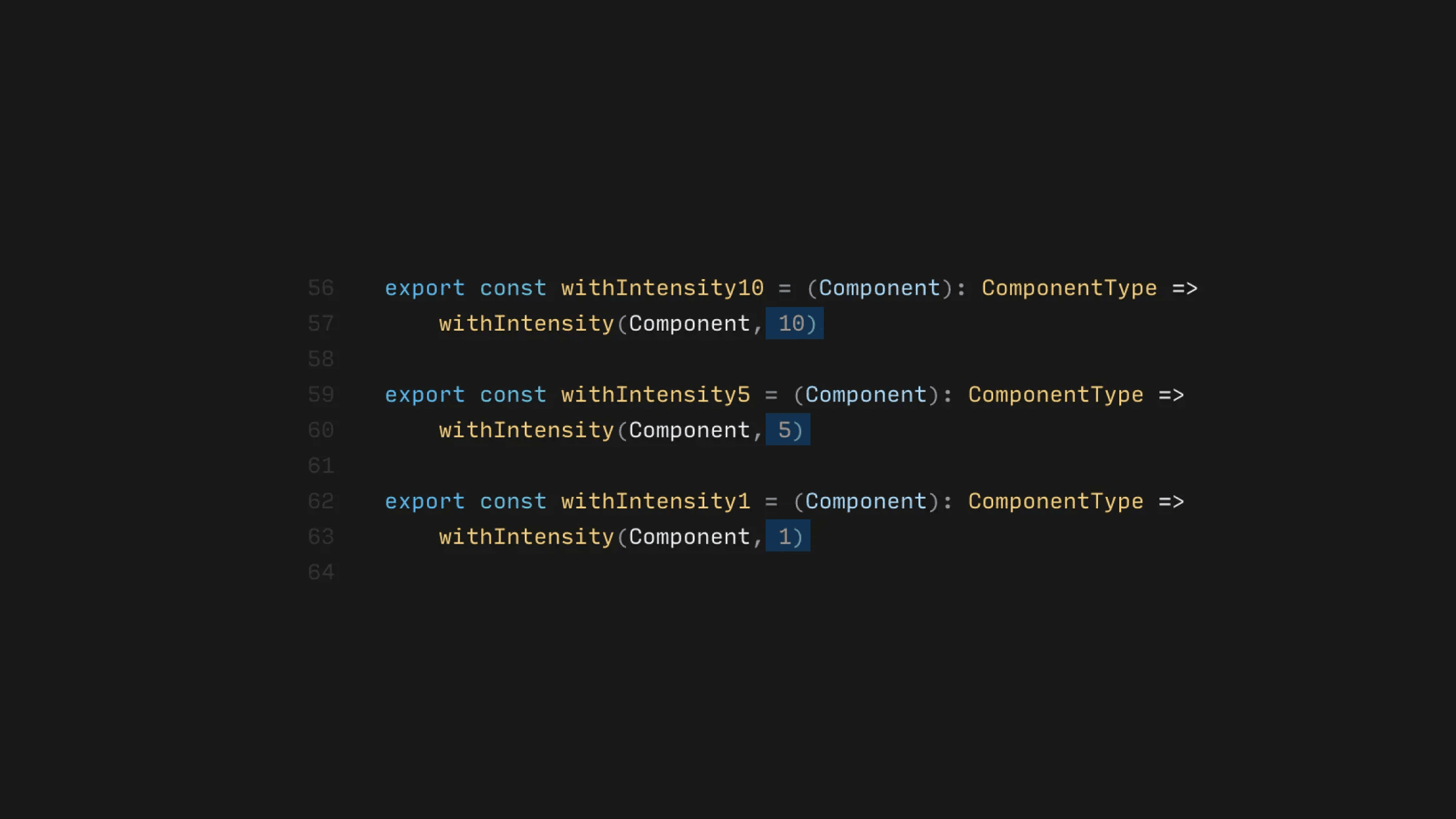
Adjusting the "look" Framer code override.
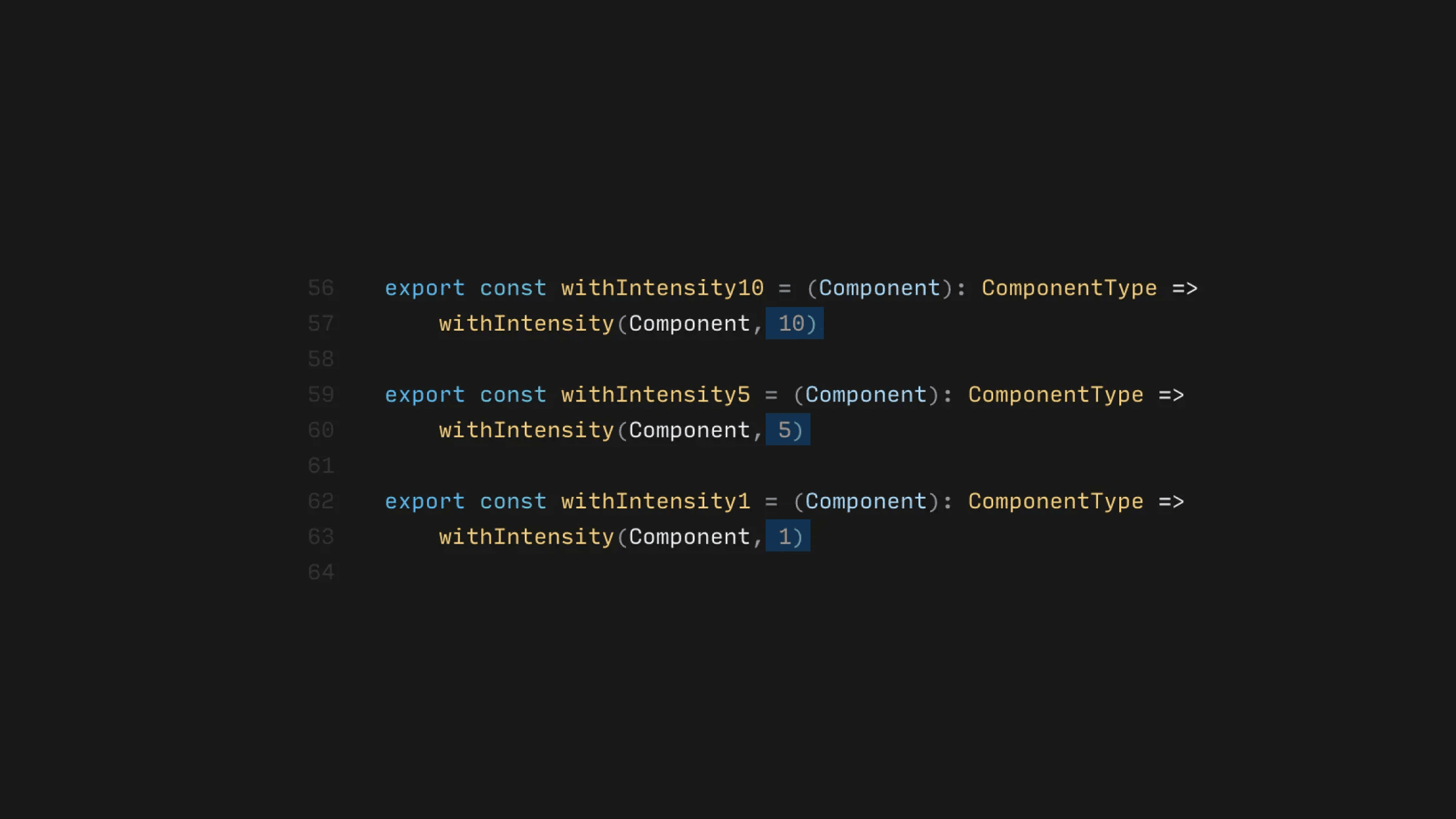
Adjusting the "look" Framer code override.
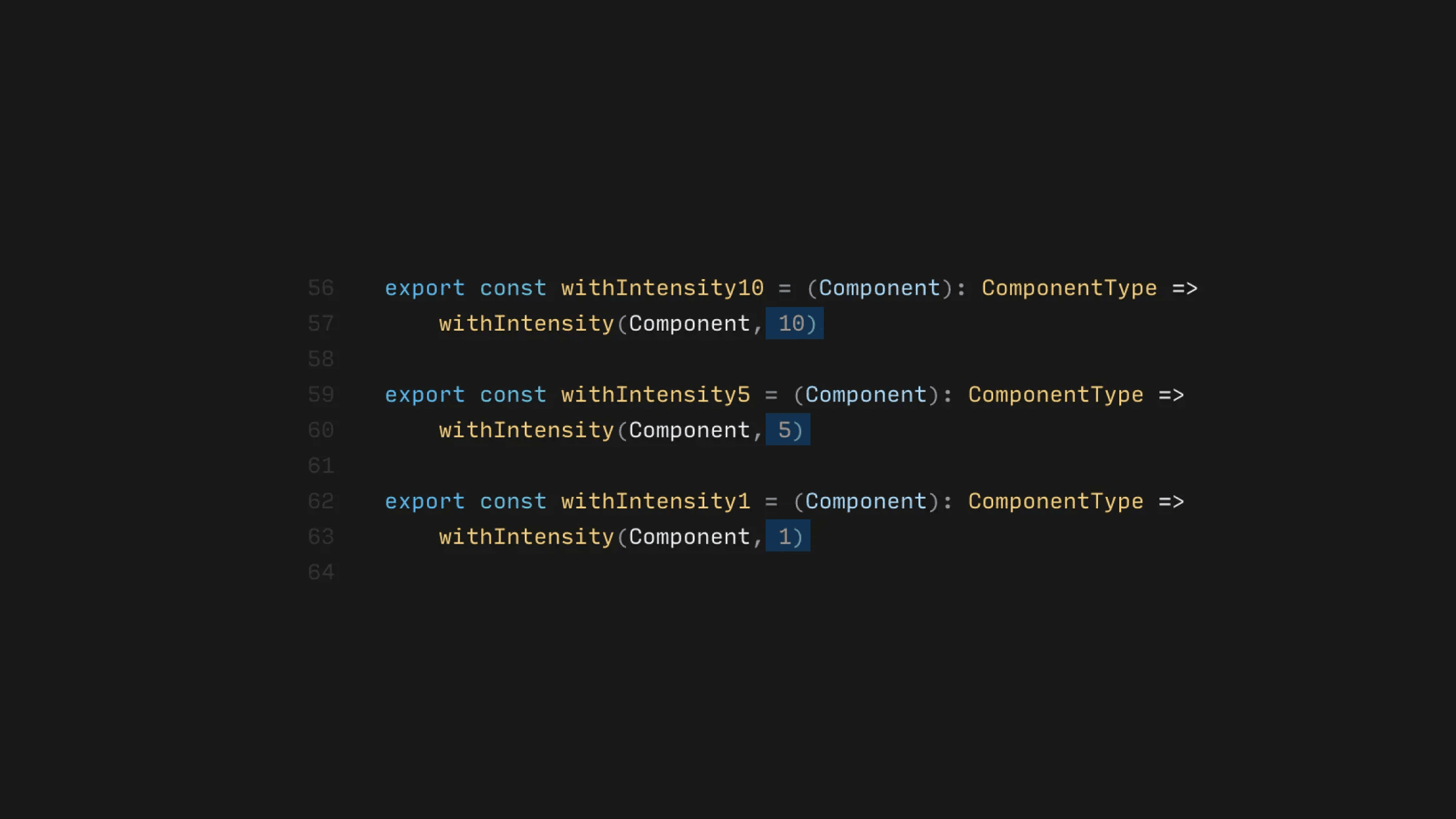
Adjusting the "look" Framer code override.
Code override for parallax hover effect
Feel free to copy the code override from below and create it for yourself in Framer from scratch.
Code override for parallax hover effect
Feel free to copy the code override from below and create it for yourself in Framer from scratch.
Code override for parallax hover effect
Feel free to copy the code override from below and create it for yourself in Framer from scratch.

import { useState, useEffect, ComponentType, useRef } from "react" import { motion, useMotionValue, useSpring, useInView } from "framer-motion" const withIntensity = (Component, maxDistancePercentage): ComponentType => { const SPRING_CONFIG = { damping: 100, stiffness: 400 } return (props) => { const x = useMotionValue(0) const y = useMotionValue(0) const ref = useRef(null) const springX = useSpring(x, SPRING_CONFIG) const springY = useSpring(y, SPRING_CONFIG) const isInView = useInView(ref) useEffect(() => { if (!isInView) { x.set(0) y.set(0) } }, [isInView]) useEffect(() => { const calculateDistance = (e) => { if (ref.current && isInView) { const rect = ref.current.getBoundingClientRect() const centerX = rect.left + rect.width / 2 const centerY = rect.top + rect.height / 2 const distanceX = e.clientX - centerX const distanceY = e.clientY - centerY x.set(distanceX * (maxDistancePercentage / 100)) y.set(distanceY * (maxDistancePercentage / 100)) } } document.addEventListener("mousemove", calculateDistance) return () => { document.removeEventListener("mousemove", calculateDistance) } }, [ref, isInView]) return ( <Component {...props} ref={ref} style={{ x: springX, y: springY, }} /> ) } } export const withIntensity10 = (Component): ComponentType => withIntensity(Component, 10) export const withIntensity5 = (Component): ComponentType => withIntensity(Component, 5) export const withIntensity1 = (Component): ComponentType => withIntensity(Component, 1)

import { useState, useEffect, ComponentType, useRef } from "react" import { motion, useMotionValue, useSpring, useInView } from "framer-motion" const withIntensity = (Component, maxDistancePercentage): ComponentType => { const SPRING_CONFIG = { damping: 100, stiffness: 400 } return (props) => { const x = useMotionValue(0) const y = useMotionValue(0) const ref = useRef(null) const springX = useSpring(x, SPRING_CONFIG) const springY = useSpring(y, SPRING_CONFIG) const isInView = useInView(ref) useEffect(() => { if (!isInView) { x.set(0) y.set(0) } }, [isInView]) useEffect(() => { const calculateDistance = (e) => { if (ref.current && isInView) { const rect = ref.current.getBoundingClientRect() const centerX = rect.left + rect.width / 2 const centerY = rect.top + rect.height / 2 const distanceX = e.clientX - centerX const distanceY = e.clientY - centerY x.set(distanceX * (maxDistancePercentage / 100)) y.set(distanceY * (maxDistancePercentage / 100)) } } document.addEventListener("mousemove", calculateDistance) return () => { document.removeEventListener("mousemove", calculateDistance) } }, [ref, isInView]) return ( <Component {...props} ref={ref} style={{ x: springX, y: springY, }} /> ) } } export const withIntensity10 = (Component): ComponentType => withIntensity(Component, 10) export const withIntensity5 = (Component): ComponentType => withIntensity(Component, 5) export const withIntensity1 = (Component): ComponentType => withIntensity(Component, 1)

import { useState, useEffect, ComponentType, useRef } from "react" import { motion, useMotionValue, useSpring, useInView } from "framer-motion" const withIntensity = (Component, maxDistancePercentage): ComponentType => { const SPRING_CONFIG = { damping: 100, stiffness: 400 } return (props) => { const x = useMotionValue(0) const y = useMotionValue(0) const ref = useRef(null) const springX = useSpring(x, SPRING_CONFIG) const springY = useSpring(y, SPRING_CONFIG) const isInView = useInView(ref) useEffect(() => { if (!isInView) { x.set(0) y.set(0) } }, [isInView]) useEffect(() => { const calculateDistance = (e) => { if (ref.current && isInView) { const rect = ref.current.getBoundingClientRect() const centerX = rect.left + rect.width / 2 const centerY = rect.top + rect.height / 2 const distanceX = e.clientX - centerX const distanceY = e.clientY - centerY x.set(distanceX * (maxDistancePercentage / 100)) y.set(distanceY * (maxDistancePercentage / 100)) } } document.addEventListener("mousemove", calculateDistance) return () => { document.removeEventListener("mousemove", calculateDistance) } }, [ref, isInView]) return ( <Component {...props} ref={ref} style={{ x: springX, y: springY, }} /> ) } } export const withIntensity10 = (Component): ComponentType => withIntensity(Component, 10) export const withIntensity5 = (Component): ComponentType => withIntensity(Component, 5) export const withIntensity1 = (Component): ComponentType => withIntensity(Component, 1)