Effect
Flashlight Effect in Framer
This is a Framer implementation of the flashlight effect from the Cred's website. Scroll down to see how you can add it to your own Framer websites.
Created by
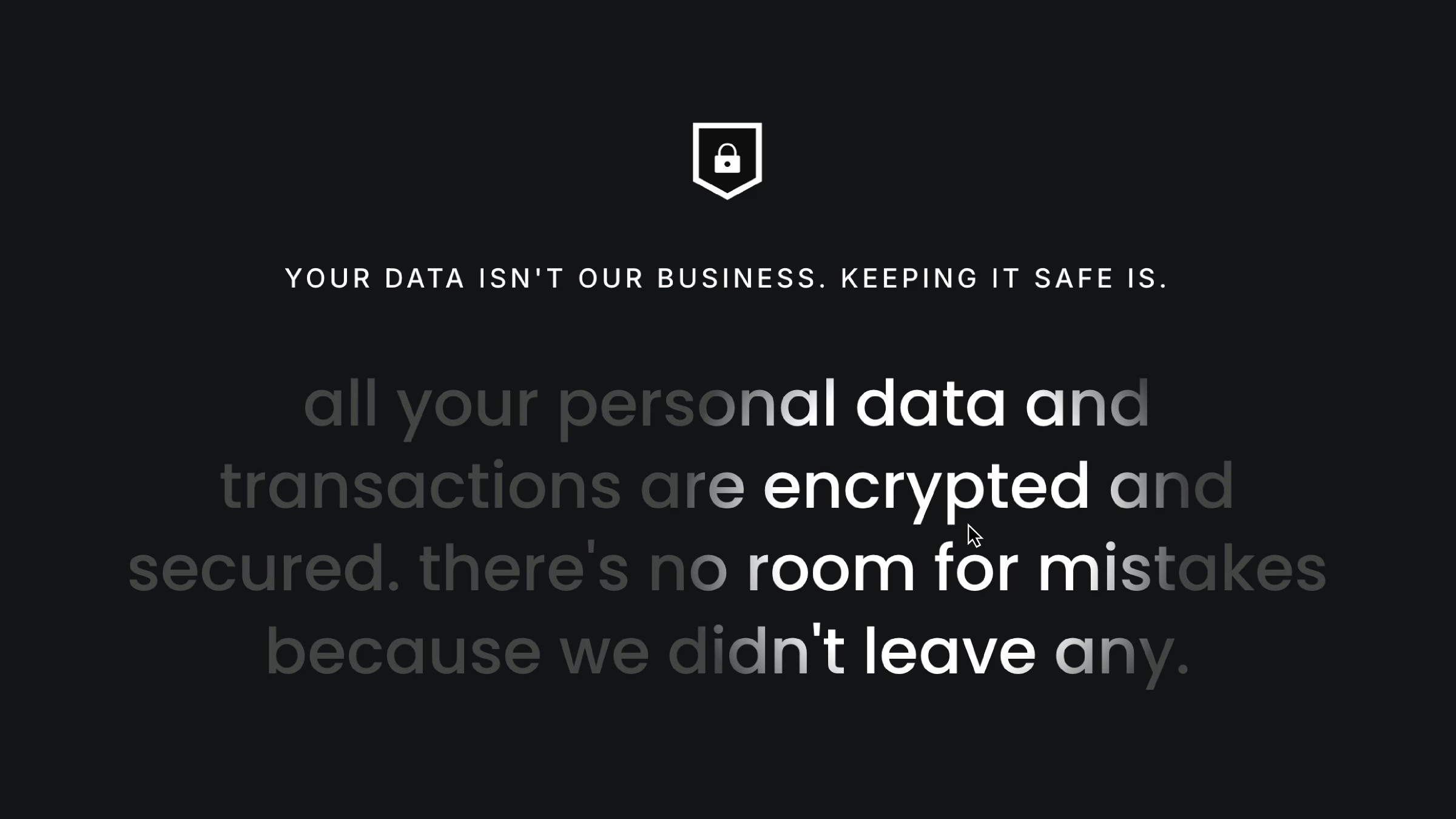
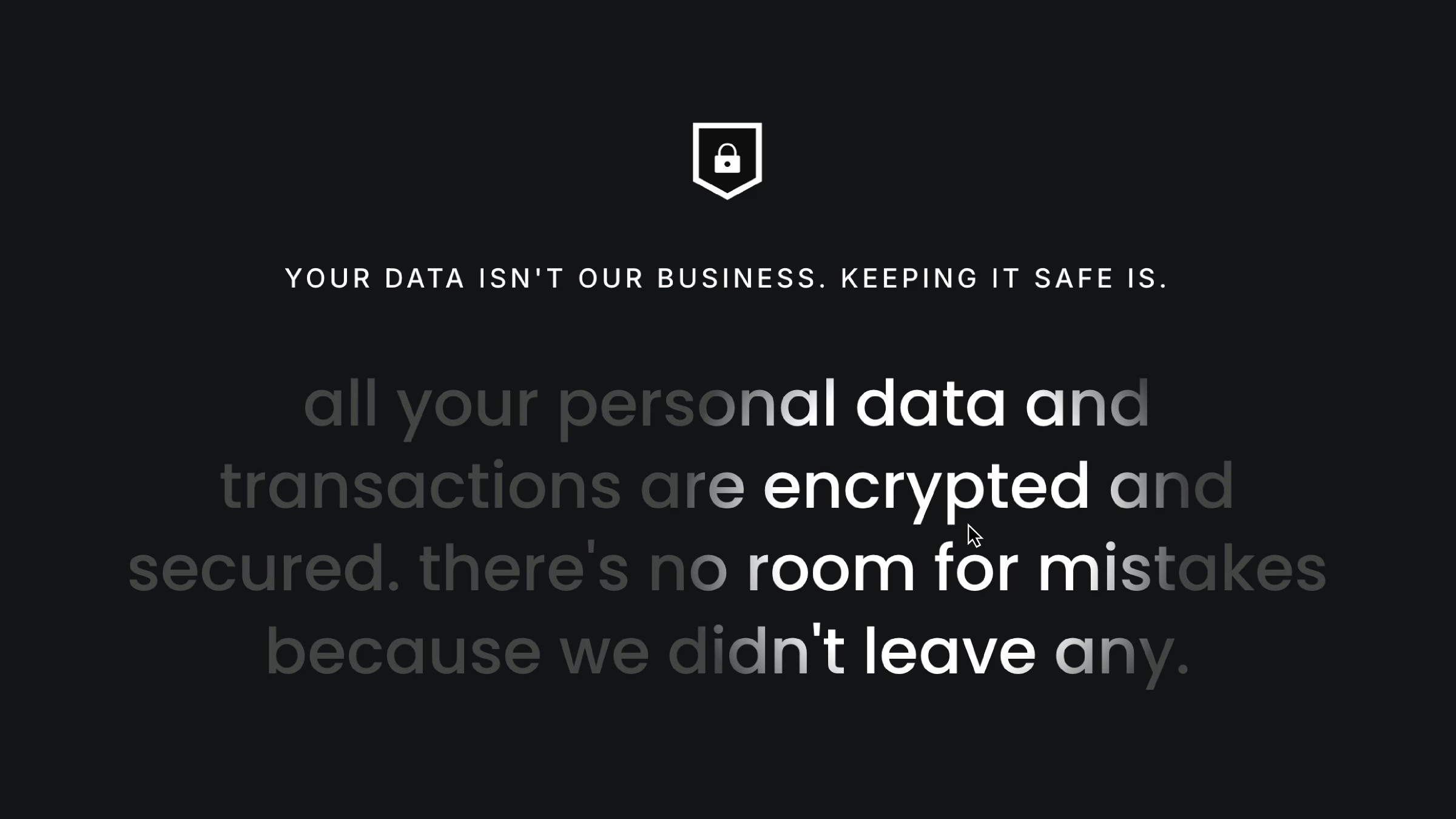
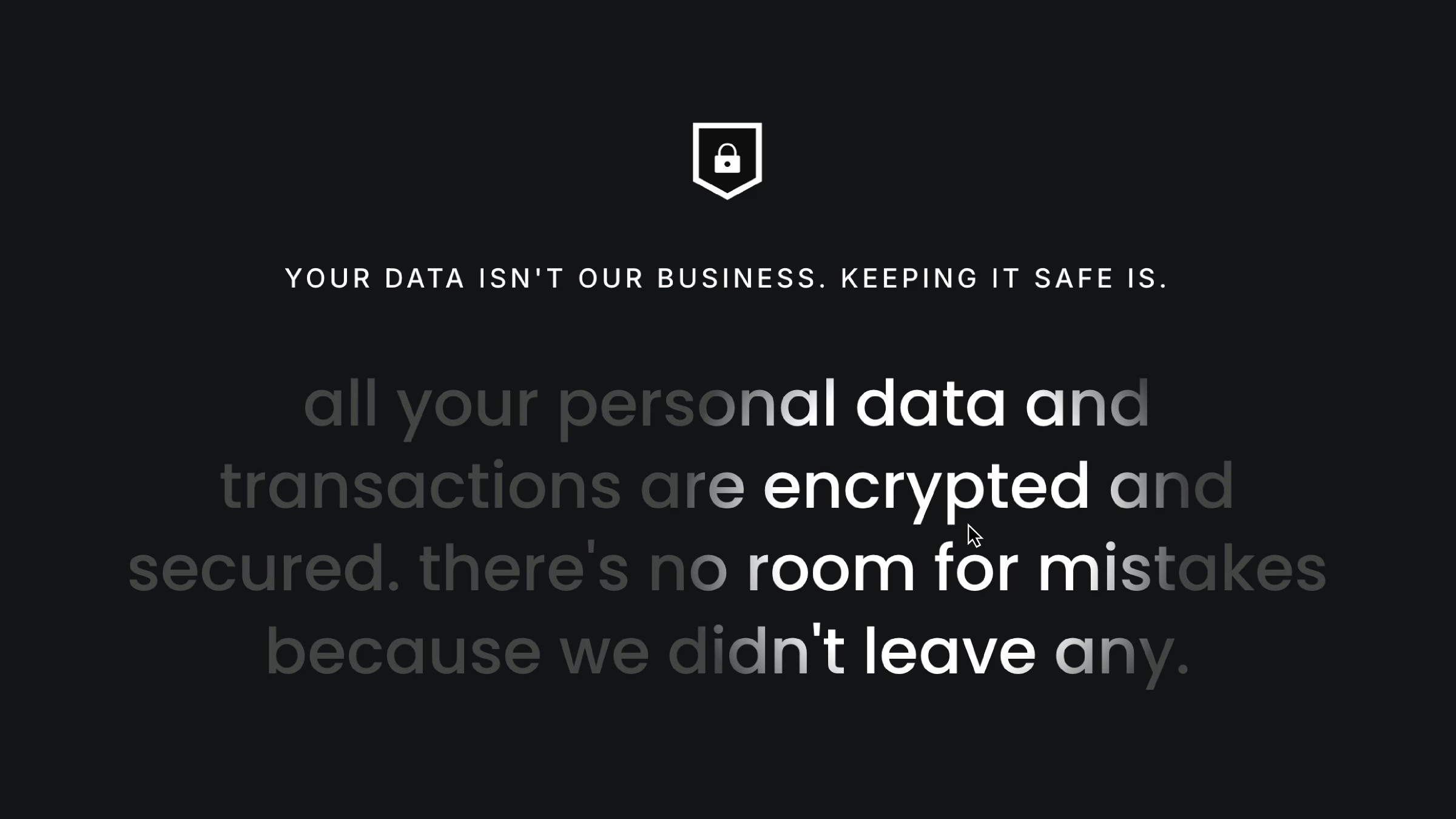
About the resource
This is a Framer code override you can apply to any layer to create the flashlight effect. The trick here is to have the exact same text layer stacked below the overridden text layer. You can easily adjust the effect by changing a few values in the code—it’s that simple!
You can tweak the "mask size" to control how far the spotlight reaches, and adjust the "duration" and "ease" of the animation.
About the resource
This is a Framer code override you can apply to any layer to create the flashlight effect. The trick here is to have the exact same text layer stacked below the overridden text layer. You can easily adjust the effect by changing a few values in the code—it’s that simple!
You can tweak the "mask size" to control how far the spotlight reaches, and adjust the "duration" and "ease" of the animation.
About the resource
This is a Framer code override you can apply to any layer to create the flashlight effect. The trick here is to have the exact same text layer stacked below the overridden text layer. You can easily adjust the effect by changing a few values in the code—it’s that simple!
You can tweak the "mask size" to control how far the spotlight reaches, and adjust the "duration" and "ease" of the animation.
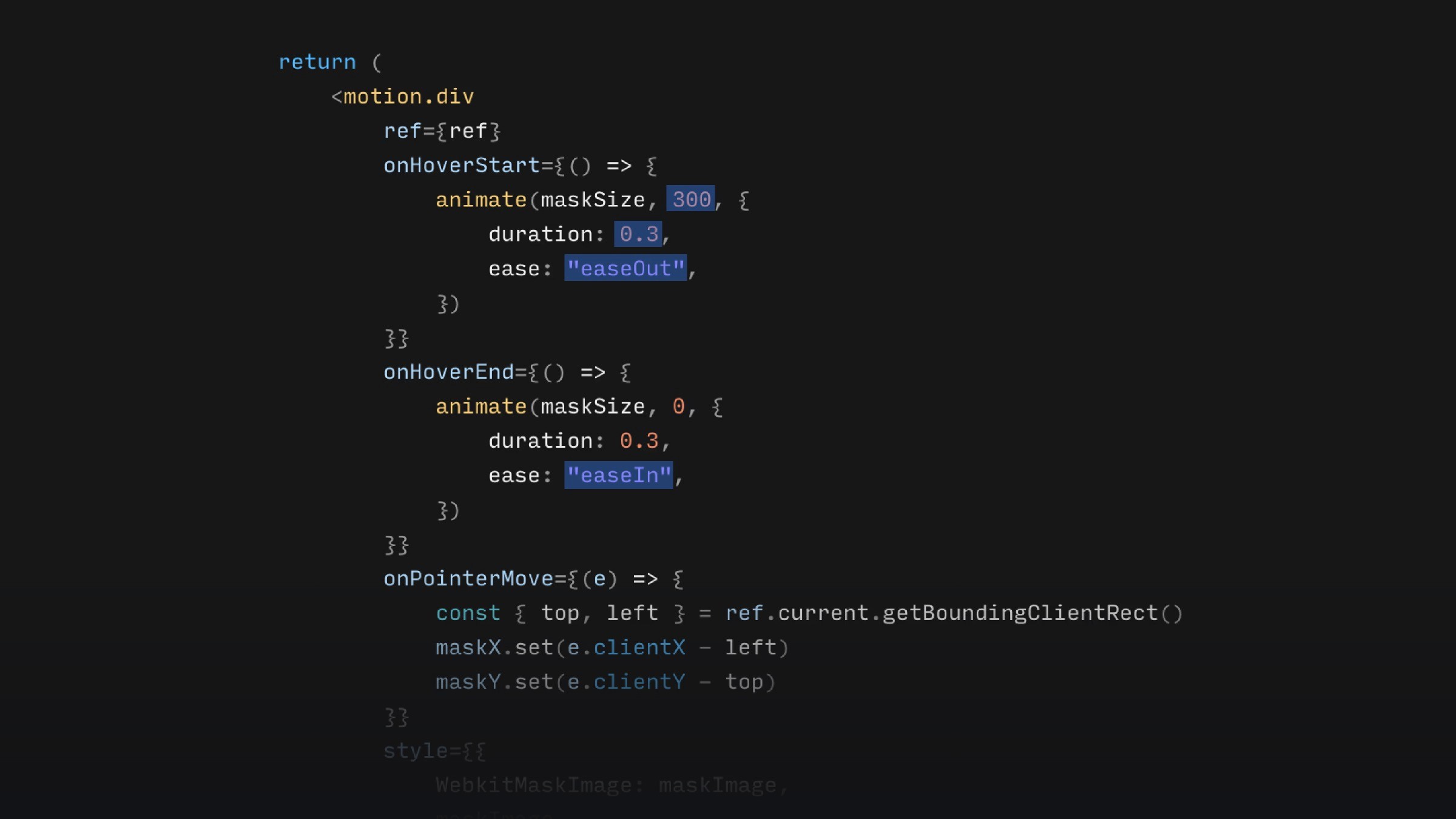
Editing the flashlight effect override in Framer.
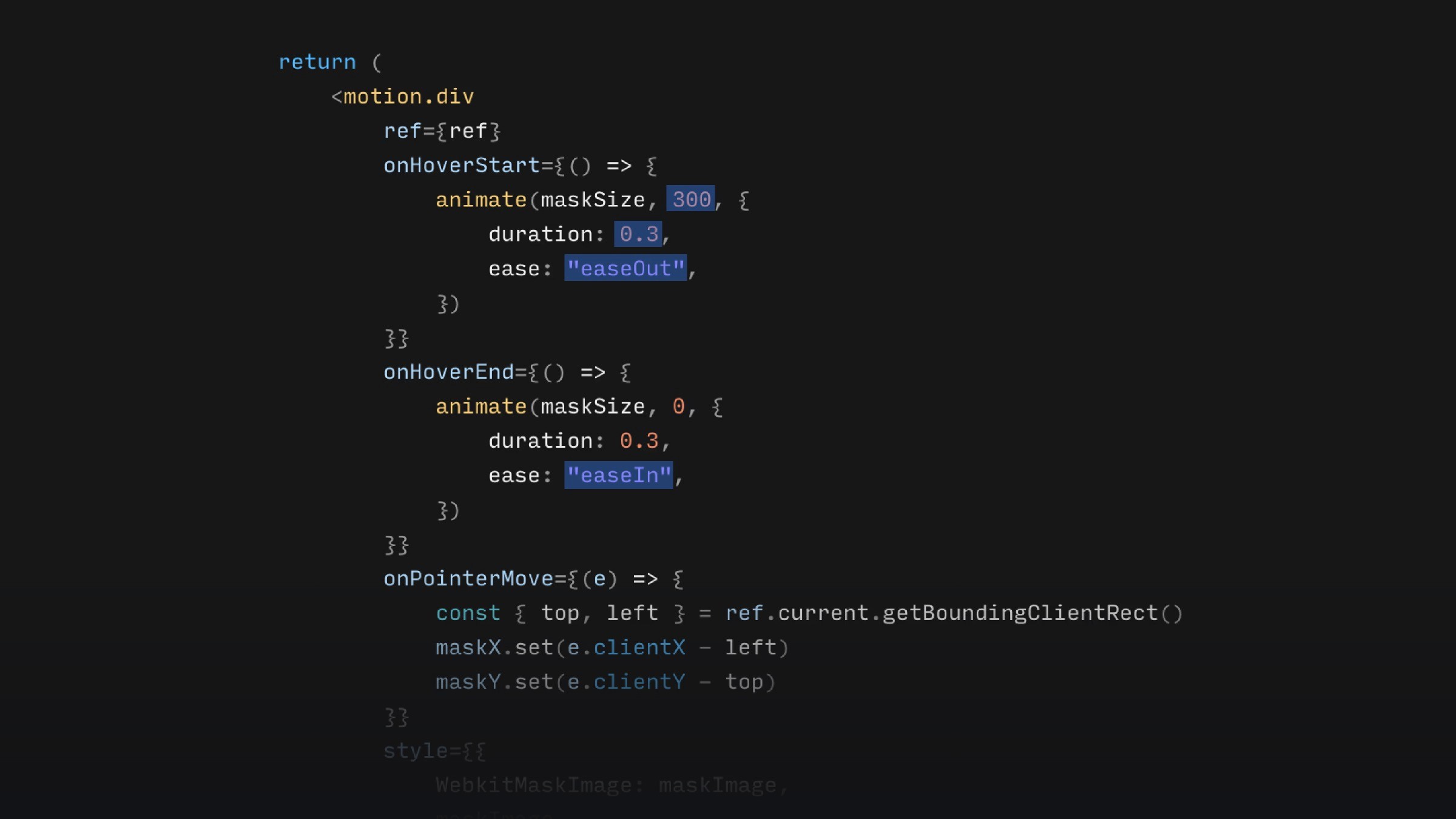
Editing the flashlight effect override in Framer.
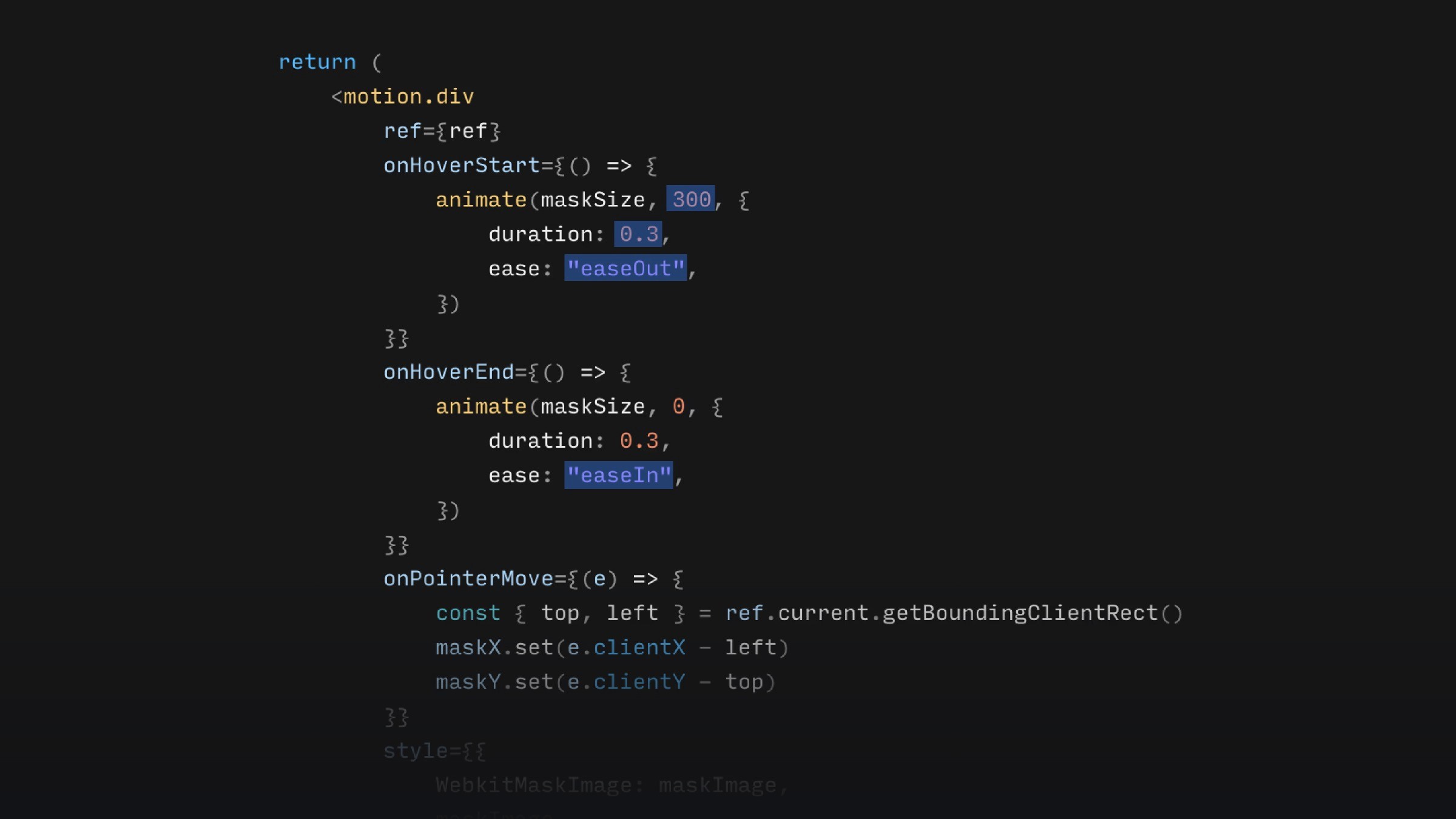
Editing the flashlight effect override in Framer.
Code Override
Create a code override in your project by going to the assets panel on the left, then scroll down to "code". Click the "+" button to create a new override. Replace the auto-generated code with the code below, press ⌘ + S to save it, and apply it to any layer.
Code Override
Create a code override in your project by going to the assets panel on the left, then scroll down to "code". Click the "+" button to create a new override. Replace the auto-generated code with the code below, press ⌘ + S to save it, and apply it to any layer.
Code Override
Create a code override in your project by going to the assets panel on the left, then scroll down to "code". Click the "+" button to create a new override. Replace the auto-generated code with the code below, press ⌘ + S to save it, and apply it to any layer.

import type { ComponentType } from "react" import { useEffect, useRef } from "react" import { motion, useMotionValue, useMotionTemplate, animate, } from "framer-motion" export function withSoftMask(Component): ComponentType { return (props) => { const maskX = useMotionValue(0) const maskY = useMotionValue(0) const maskSize = useMotionValue(0) const maskImage = useMotionTemplate`radial-gradient(circle ${maskSize}px at ${maskX}px ${maskY}px, black, black 50%, transparent 80%)` const ref = useRef(null) return ( <motion.div ref={ref} onHoverStart={() => { animate(maskSize, 300, { duration: 0.3, ease: "easeOut", }) }} onHoverEnd={() => { animate(maskSize, 0, { duration: 0.3, ease: "easeIn", }) }} onPointerMove={(e) => { const { top, left } = ref.current.getBoundingClientRect() maskX.set(e.clientX - left) maskY.set(e.clientY - top) }} style={{ WebkitMaskImage: maskImage, maskImage, WebkitMaskSize: "100%", maskSize: "100%", WebkitMaskComposite: "destination-out", maskComposite: "destination-out", }} {...props} > <Component {...props} /> </motion.div> ) } }

import type { ComponentType } from "react" import { useEffect, useRef } from "react" import { motion, useMotionValue, useMotionTemplate, animate, } from "framer-motion" export function withSoftMask(Component): ComponentType { return (props) => { const maskX = useMotionValue(0) const maskY = useMotionValue(0) const maskSize = useMotionValue(0) const maskImage = useMotionTemplate`radial-gradient(circle ${maskSize}px at ${maskX}px ${maskY}px, black, black 50%, transparent 80%)` const ref = useRef(null) return ( <motion.div ref={ref} onHoverStart={() => { animate(maskSize, 300, { duration: 0.3, ease: "easeOut", }) }} onHoverEnd={() => { animate(maskSize, 0, { duration: 0.3, ease: "easeIn", }) }} onPointerMove={(e) => { const { top, left } = ref.current.getBoundingClientRect() maskX.set(e.clientX - left) maskY.set(e.clientY - top) }} style={{ WebkitMaskImage: maskImage, maskImage, WebkitMaskSize: "100%", maskSize: "100%", WebkitMaskComposite: "destination-out", maskComposite: "destination-out", }} {...props} > <Component {...props} /> </motion.div> ) } }

import type { ComponentType } from "react" import { useEffect, useRef } from "react" import { motion, useMotionValue, useMotionTemplate, animate, } from "framer-motion" export function withSoftMask(Component): ComponentType { return (props) => { const maskX = useMotionValue(0) const maskY = useMotionValue(0) const maskSize = useMotionValue(0) const maskImage = useMotionTemplate`radial-gradient(circle ${maskSize}px at ${maskX}px ${maskY}px, black, black 50%, transparent 80%)` const ref = useRef(null) return ( <motion.div ref={ref} onHoverStart={() => { animate(maskSize, 300, { duration: 0.3, ease: "easeOut", }) }} onHoverEnd={() => { animate(maskSize, 0, { duration: 0.3, ease: "easeIn", }) }} onPointerMove={(e) => { const { top, left } = ref.current.getBoundingClientRect() maskX.set(e.clientX - left) maskY.set(e.clientY - top) }} style={{ WebkitMaskImage: maskImage, maskImage, WebkitMaskSize: "100%", maskSize: "100%", WebkitMaskComposite: "destination-out", maskComposite: "destination-out", }} {...props} > <Component {...props} /> </motion.div> ) } }